"
Repository
Memorized Word Application with Alertify.js
What Will I Learn?
- You will learn create notifications and alerts using
alertify.js
- You will learn to use
localStorage
- You will learn a
guid
function to create a unique value. - You will learn how to use the
Math.Random()
function - You will learn how to use the
setInterval()
function
Requirements
Difficulty
- Intermediate
Tutorial Contents
In this article I will tell you how to do word memorization application using notifications and alerts in alertify.js
.
If I have to talk about the application I plan to do:
The application will consist of two parts. In the first part, the end users will write the words they want to memorize in the system and in the second part application will remind them of these words at certain intervals.
You will learn how to write localStorage
events and guid
creation code with javascript in addition to the main topic in this article.
I will also use and explain the functions Math.random()
and setInterval()
in the javascript.
Let's start to build the application step by step.
- Install and include alertify.js
- Design the page
- Create guid
- Add words to system
- Create interval function and use it
- Change alert and notification themes
STEP 1: Install and include alertify.js
The alertify.js
library using npm
, we can download the folder where your files are located.
- command and download alertify.js
npm install alerifyjs --save
We will also be using jquery to perform our operations with jquery.
npm install --save jquery
So the node_modules
folder is created and all the files needed for alertify.js and jquery are in this folder.
Now include files needed for notifications and alerts and I will also include the bootstrap cdn
to use the bootstrap theme.
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<link href="https://stackpath.bootstrapcdn.com/bootstrap/4.1.2/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-Smlep5jCw/wG7hdkwQ/Z5nLIefveQRIY9nfy6xoR1uRYBtpZgI6339F5dgvm/e9B" crossorigin="anonymous">
<link rel="stylesheet" href="./node_modules/alertifyjs/build/css/alertify.min.css" />
<link rel="stylesheet" href="./node_modules/alertifyjs/build/css/themes/default.css" />
<script src="./node_modules/jquery/dist/jquery.min.js"></script>
<script src="./node_modules/alertifyjs/build/alertify.min.js"></script>
<script src="script.js"></script>
<title>Alertify</title>
</head>
I will write my JavaScript code in the script.js file.
STEP 2: Design the page
I would place a bootstrap jumbotron
on the page. There will be two buttons in this area, the first button to start learning and the second button will change the theme.
Just below the jumbotron, there will be a area to add words to memorize on the left side of the page, and a list of these words will appear on the right side of the page.
<body>
<div class="container">
<div class="jumbotron">
<div class="row">
<div class="col-md-6">
<button type="button" id="btnLearn" class="btn btn-primary">Start Learning</button>
</div>
<div class="col-md-6">
<button type="button" id="btnTheme" class="btn btn-primary">Change Theme</button>
</div>
</div>
</div>
<div class="row">
<div class="col-md-6">
<form>
<div class="form-group">
<label >Word :</label>
<input type="text" class="form-control" id="inputWord" placeholder="Enter reminder word">
</div>
<hr/>
<button type="button" id="btnWord" class="btn btn-primary">Submit</button>
</form>
</div>
<div class="col-md-6">
<ul class="list-group" id="ulWord">
</ul>
</div>
</div>
</div>
</body>
I have defined the <ul>
tag to show the word list, but have not yet defined the <li>
tags because will add the <li>
tag with jquery when the words are added.
Screenshot 1
STEP 3: Create guid
I will keep the added word in an object and set the id
and name
property of that object.
I will define a guid value for the id attribute, so I will separate the objects from each other and hold the added word in the name property.
I'll first define a function to create a guid value, and if I need guid value, I will need to execute this function.
Now let's create this function in the script.js file and then explain what we do.
function generateID() {
var time = new Date().getTime();//find time
return 'oooooooo-oooo-4ooo-kooo-oooooooooooo'.replace(/[ok]/gi, function (c) {//set character for guid
var random = (time + Math.random() * 16) % 16 | 0;//set hexadecimal
time = Math.floor(time / 16);
return (c === 'o' ? random : (random & 0x3 | 0x8)).toString(16);//Write random character instead of 'o' character
});
}
The first thing I do in this function is to find the time of the moment because I want it to be unique.
The value should be a certain number of characters and strictly 15th characters should be 4, so I'm writing to give 36 characters, I will replace all o
those characters with a random character.
I will use the replace()
function for the replacement. I have to go beyond the standard work of the replace() function because will replace all those o
and k
with the new value.
The reason why I use gi
in the first parameter of the replace () function is /[ok]/
all the o and k change are for changing the second parameter without upper case the lower case.
Then I use the time variable to find a random value, and replace the random value with the hexadecimal equivalent of all o
and k
.
I'm creating a random value using the Math.random()
function, and since this function produces a value between 0 and 1, I multiply it by 16 to be hexadecimal.
Now that we have the guid creation function, let's see what kind of result it will produce.
console.log(generateID());
Screenshot 2
As you can see a different value was produced each time.
STEP 4: Add words to system
When we click the submit button, we read the value in the input and send it to an object but let's ask if you are sure using alertify in the beginning.
We need to use the alertify.confirm()
function because our process passes through a confirmation process.
If the OK
button is clicked, we transfer the word to the object and push that object into a predefined array.
We send this array to localStorage with localStorage.setItem()
.
The localStorage setItem() function takes two parameters. The first parameter is the key
of the value we want to store, and the second parameter is the value
.
One property that should not be forgotten is that in localStorage, only string-type values can be stored. We need to use the JSON.stringify()
function here because we want to store a json-type array.
Then print this array on the screen and notify the user using alertify notification.
I will use the alertify.success()
function when the OK button is clicked, and if I click on the cancel button, I will use alertify.error()
.
$('#btnWord').click(function(){
alertify.confirm('Add Word', 'Do you add the word', function(){ //if you want to add
var word={
id:generateID(),
name:$('#inputWord').val()
}
words.push(word);
localStorage.setItem('word',JSON.stringify(words));
addWordToList();
alertify.success('Word added')
}, function(){ //if you do not want to add
alertify.error('Word did not add')
});
})
Screenshot 3
When Submit
button clicked
Screenshot 4
When OK
button clicked
Screenshot 5
When CANCEL
button clicked
Create the function addWordToList()
.
function addWordToList(){
var wordArray=JSON.parse(localStorage.getItem('word'));//json parsing localStorage data
$( "#ulWord" ).empty();//clear
tag
wordArray.forEach(element => {
$('#ulWord').append(''+element.name+'')//Write the data to the tag
});
}
Here we will write the words to the <li>
tag, but we need to access the array at localStorage before.
We use the getItem()
method to read data from localStorage and we do need to enter the key value. We need to use the JSON.parse()
function in order to be able to read the localStorage data because it is of type json.
After accessing the data in localStorage, we return with forEach
between the data and write to the screen using append()
function but before, we use the empty()
function we need to clear the <ul>
tag so we can get rid of the data again.
Screenshot 6
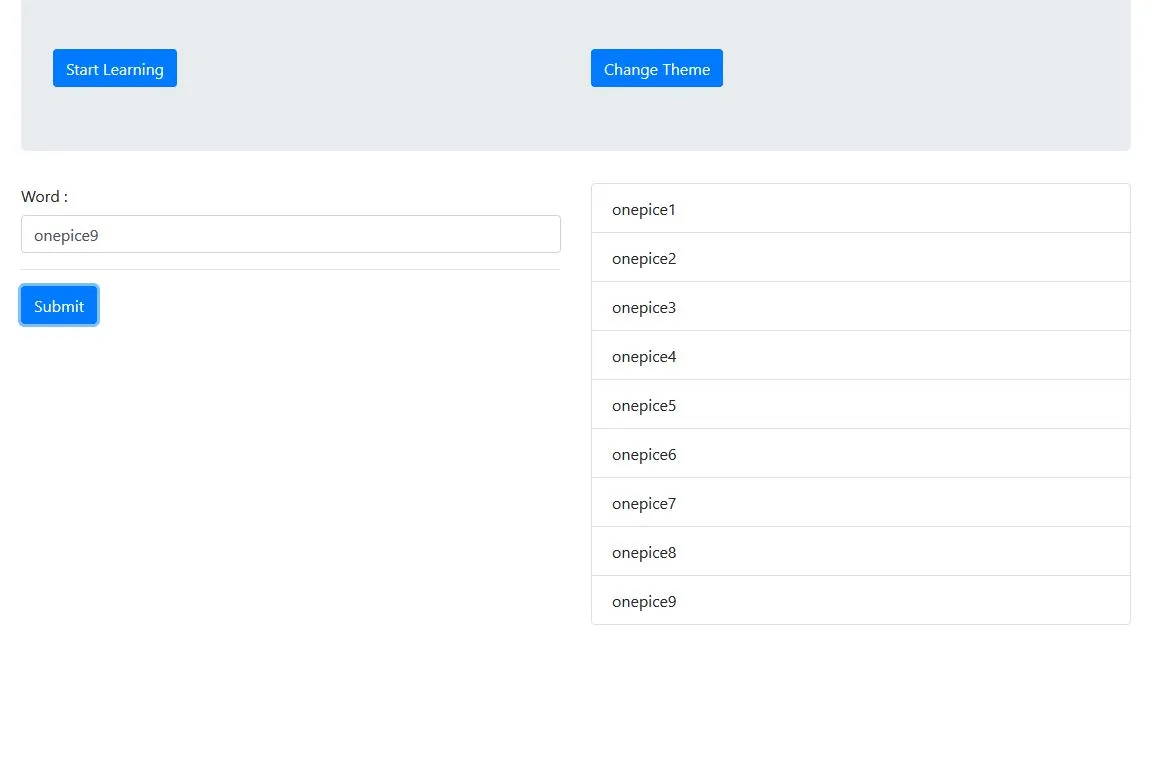
STEP 5: Create interval function and use it
Let's create a function named interval and define the setInterval()
function that runs certain code at specific time intervals.
In the setInterval() function, we create a random number between 0 and array lenght in the localStorage and use this random number as the array index so that we can show a word entered at random.
Using the alertify.notify()
function, we can set both the theme and the duration of the notifications.
If you set the duration to 0
, it will remain on the screen until you click on the notification.
After clicking on it, we will issue an alert to the screen asking for input and ask them to write the learned word with this alert.
The alertify.prompt()
function is used and the value can be captured in this operation.
function interval() {
setInterval(function(){
var wordArray=JSON.parse(localStorage.getItem('word'));//json parsing localStorage data
var index = Math.floor((Math.random() * wordArray.length));//create random index
//console.log(wordArray[index].name);
alertify.notify(wordArray[index].name, 'warning', 0, function(){ //show up until clicked on with warning notification
alertify.prompt( 'Word', 'What is the last learned word','', function(evt, value) { // input from end user
if(value==wordArray[index].name){//if the value entered is correct
alertify.success('True');
}else{//if the value entered is wrong
alertify.error('False, True word is '+wordArray[index].name) ;
}
}
, function() {//If the cancel button is clicked
alertify.error('Cancel')
});
});
}, 3000);//millisecond
}
Let's run this interval() function after clicking the start learning button.
$('#btnLearn').click(function(){
interval();
});
Screenshot 7
Words
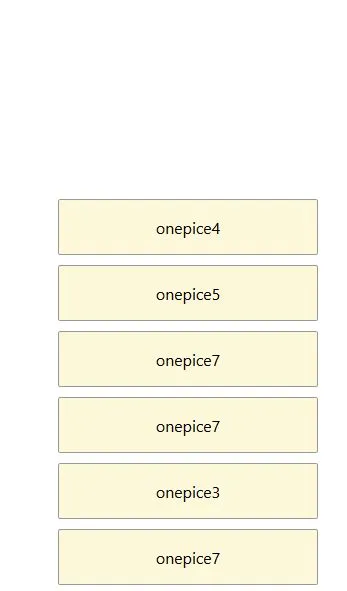
Screenshot 8
When clicked
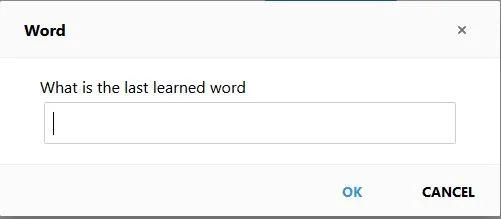
STEP 6: Change alert and notification themes
We can change the appearance of notifications and alerts when the change theme button is clicked.
When we set the css class to alertify.defaults
, the alerts and notifications will now change accordingly.
We can create our own classes or use ready-made classes.
Let's set the classes of the buttons as bootstrap classes.
$('#btnTheme').click(function(){
//override defaults
alertify.defaults.transition = "slide";
alertify.defaults.theme.ok = "btn btn-success";
alertify.defaults.theme.cancel = "btn btn-danger";
alertify.defaults.theme.input = "form-control";
});
Screenshot 9
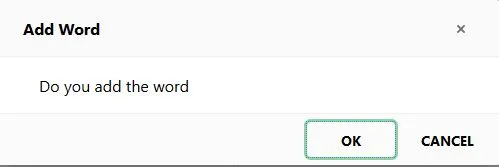
Screenshot 10
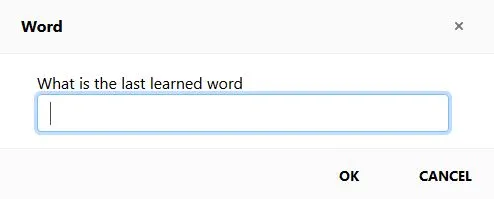
Proof of Work Done
https://github.com/onepicesteem/memorized-word-application-with-alertify.js