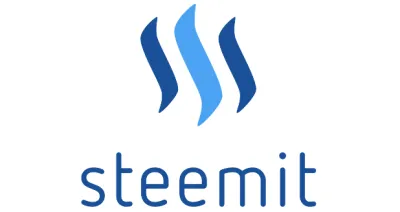
Introduction
This tutorial will show you how to automatically follow users with a bot.
First, you will need to set-up a NodeJS development environment. You can follow along the tutorial at https://steemit.com/javascript/@money-dreamer/nodejs-steemit-bot-tutorial if you need to. That tutorial is a pre-requisite for this tutorial unless if you are already familiar with JavaScript, NodeJS, GIT and the Steemit API.
You can view the full source code at: https://github.com/AdamCox9/Steemit-Follow-Bot
After getting your development environment set-up, copy and paste this code into a file named follow_trending_authors.js
and execute it like node follow_trending_authors.js
from a terminal as described in the previous tutorial. This will bring in all the functions from the Steem library, set the user/pass and create a log-in session to the Steem blockchain. The followingArray saves the new account so no duplicate following attempts.
var steem = require('steem');
var username = 'ENTER_USERNAME_HERE';
var password = 'ENTER_PASSWORD_HERE';
var wif = steem.auth.toWif(username, password, 'posting');
var followingArray = [];
Let's get the trending categories in our initial call. You can view the data printed to the console to become familiar with it.
steem.api.getState('/trending', function(err, result) {
console.log(err, result);
...
});
The sleep
function will time out the script so it does not make requests too fast:
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
Create a function that will loop over the accounts in the trending tag:
async function followAccountsInTrending(result) {
var content = result.content;
var accounts = result.accounts;
for(var attributename in accounts) {
//console.log(attributename+': '+accounts[attributename]);
var following = accounts[attributename].name;
let followReq = ['follow']
followReq.push({follower: username, following: following, what: ['blog']})
const customJson = JSON.stringify(followReq)
console.log( followReq );
followingArray.indexOf(following) === -1 ?
followingArray.push(following) : console.log('This item already exists');
steem.broadcast.customJsonAsync(wif, [], [username], 'follow', customJson)
.then(console.log)
.catch(console.log)
await sleep(30000);
console.log( followingArray );
}
}
Create a function that loops over each category:
async function startFollowAccountsInTrending(result) {
var trending_tags = result.tag_idx.trending;
for(var attributename in trending_tags) {
console.log(attributename+': '+trending_tags[attributename]);
steem.api.getState('/trending/'+trending_tags[attributename], function(err, result) {
followAccountsInTrending(result);
});
await sleep(30000);
}
}
Initialize the function to loop through the categories:
startFollowAccountsInTrending(result);
Further Development
It will allow you to set parameters to select who to follow. Some of these parameters will be:
- SBD/STEEM Balance
- Reputation
- Number of Followers
- Number of Following
- Total Author Rewards
- Total Curation Rewards
You can view the full source code at: https://github.com/AdamCox9/Steemit-Follow-Bot
Proof of Work:
Posted on Utopian.io - Rewarding Open Source Contributors