What Will I Learn?
A. In this tutorial I would like to show you how to work with input fields in AngularJs
B. You will use the ng-model and ng-change directives and the AngularJs watch function $watch
C. input fields in AngularJS
Requirements
Basic Knowledge of HTML and CSS language .
Basic Knowledge of JavaScript language .
Has an editor text support HTML and JavaScript .
Difficulty
Basic
In this tutorial I would like to show you how to work with input fields in AngularJs. You will use the ng-model and ng-change directives and the AngularJs watch function $watch.
Let’s say we have a form with one input field. If I type in a number the output is multiplied by itself. The form looks like this:
Number: 3
Your number multiplied by itself is: 9
This is the index.html:
<!DOCTYPE html>
<html data-ng-app="myApp">
<head>
<script type="text/javascript" src="angular.min.js"></script>
<script type="text/javascript" src="app.js"></script>
</head>
<body>
<form data-ng-controller="NumberCtrl">
<p>Number: <input data-ng-model="number.value" />p>
<p>Your number multiplied by itself is: {{number.value * number.value}}</p>
<form>
</body>
</html>
This is the app.js:
var app = angular.module('myApp', []);
app.controller('NumberCtrl', function($scope) {
$scope.number = {
value: 0
};
});
The directive data-ng-model names the property which holds the value of the input field. The property is in the $scope of the controller. Notice that you can also use nested properties like in this case number.value.
Each time you type in a number the output gets updated immediately because of the 2-way data-binding. You change something in the GUI (input field) the model will be updated. When the model is updated the GUI will be updated (output).
Notice that we have the logic of multiplying our number in the view. Good practice is to move logic to the controller. What we can use here is the data-ng-change directive. Everytime we type in something in the input field we call a function that does the multiplication. The result is stored in a separate property which we output. Here is the changed source code.
<!DOCTYPE html>
<html data-ng-app="myApp">
<head>
<script type="text/javascript" src="angular.min.js"></script>
<script type="text/javascript" src="app.js"></script>
</head>
<body>
<form data-ng-controller="NumberCtrl">
<p>Number: <input data-ng-change="multiplyByItself()" data-ng-model="number.value" />p>
<p>Your number multiplied by itself is: {{number.multipliedByItself}}</p>
<form>
</body>
</html>
var app = angular.module('myApp', []);
app.controller('NumberCtrl', function($scope) {
$scope.number = {
value: 0,
multipliedByItself: 0
};
$scope.multiplyByItself = function() {
$scope.number.multipliedByItself = $scope.number.value * $scope.number.value;
}
});
The problem here is that number.multipliedByItself in the output will only be updated when you type in a number in the input field. Only then the multiply function will be executed by data-ng-change. What happens if the property number.value is changed by another controller and not by typing in a number in the input field? number.multipliedByItself won’t be updated because the data-ng-change function will never be called.
What we can do here is use AngularJs $scope.$watch and get rid of data-ng-change:
<!DOCTYPE html>
<html data-ng-app="myApp">
<head>
<script type="text/javascript" src="angular.min.js"></script>
<script type="text/javascript" src="app.js"></script>
</head>
<body>
<form data-ng-controller="NumberCtrl">
<p>Number: <input data-ng-model="number.value" />p>
<p>Your number multiplied by itself is: {{number.multipliedByItself}}</p>
<form>
</body>
</html>
var app = angular.module('myApp', []);
app.controller('NumberCtrl', function($scope) {
$scope.number = {
value: 0,
multipliedByItself: 0
};
var multiplyByItself = function() {
$scope.number.multipliedByItself = $scope.number.value * $scope.number.value;
}
$scope.$watch('number.value', multiplyByItself);
});
$scope.$watch takes a string as first argument which represents the property. Everytime the property value changes the second argument which is a function will be called. That means that if you type in something in the input field the property number.value changes so the function multiplyByItself is called which updates the output property. If another controller or anything else changes number.value the output property will also automatically be updated.
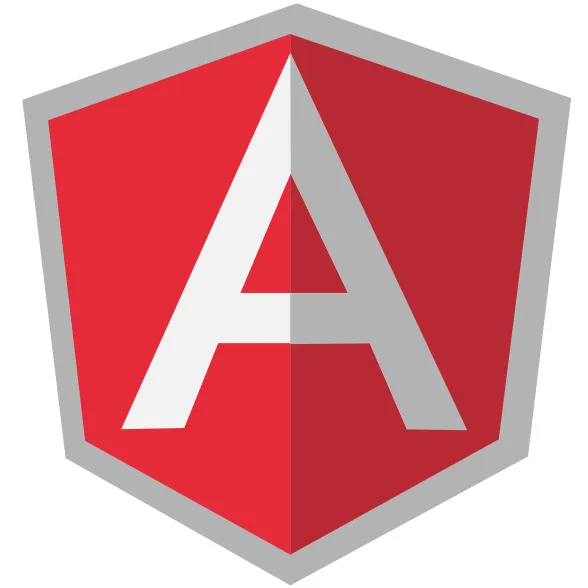
Posted on Utopian.io - Rewarding Open Source Contributors