I am a newbie on the programming world and I am trying to learn Python as it is the easiest and very strong programming language. I've learnt all basic theories and the programming syntax and then I have solved some problems from urionlinejudge. Now I'am trying to learn GUI Appliations and I am trying to learn Tkinter module of Python.
Previously I posted a Leap Year Checker GUI application that I made with Python and Tkinter module of python. I made another GUI which is a Restaurant Management System.
In this project, there are two parts. In the first part, I put the food functionalities and in the second part, I put the calculator functionalities. I have divided this blog into two parts for betterment. I will post the second part after a few days where there will be a calculator.
Let us jump to the codes.
Importing, Naming and Sizing
import tkinter as tk
import datetime
from tkinter import messagebox
date1 = datetime.date.today()
win = tk.Tk()
win.title('Restaurant Management System')
win.geometry('850x700+300+5')
win.resizable(0,0)
food functions
# ------------------ food functions ------------------
def count_price():
biriyani_number = biriyani_entry.get()
kacchi_number= kacchi_entry.get()
burger_number = burger_entry.get()
coffee_number = coffee_entry.get()
cold_coffee_number = cold_coffee_entry.get()
tea_number = tea_entry.get()
mineral_water_number = mineral_water_entry.get()
try:
price_number = (int(biriyani_number)*230)+(int(kacchi_number)*260)+(int(burger_number)*190)+(int(coffee_number)*60)+(int(cold_coffee_number)*130)+(int(tea_number)*20)+(int(mineral_water_number)*15)
price_entry.delete(0,'end')
price_entry.insert(0, (str(price_number)+' '+'TAKA'))
vat_entry.delete(0,'end')
vat_entry.insert(0,'00 TAKA')
discount_entry.delete(0,'end')
discount_entry.insert(0,'00 TAKA')
total_price_entry.delete(0,'end')
total_price_entry.insert(0,(str(price_number)+' '+ 'TAKA'))
except:
tk.messagebox.showerror('Error', 'Please enter valid numbers in all the fields.')
frames
top = tk.Frame(win,height=150 ,bg='light blue')
top.pack(fill=tk.BOTH)
bottom = tk.Frame(win, height=500, bg='white')
bottom.pack(fill=tk.BOTH, expand=True)
heading
heading = tk.Label(top, text='Jashim Tea Stall and Restaurant', bg='light blue', font='arial 17 bold')
heading.pack()
today_date = tk.Label(top, text=f'Date: {date1}', bg='light blue', font='arial 14 bold')
today_date.pack()
labels
biriyani_label = tk.Label(bottom, text='Biriyani: ', font='arial 14 bold', bg='white')
biriyani_label.place(x=30, y=20)
kacchi_label = tk.Label(bottom, text='Kacchi: ', font='arial 14 bold', bg='white')
kacchi_label.place(x=30, y=100)
burger_label = tk.Label(bottom, text='Burger: ', font='arial 14 bold', bg='white')
burger_label.place(x=30, y=180)
coffee_label = tk.Label(bottom, text='Coffee: ', font='arial 14 bold', bg='white')
coffee_label.place(x=30, y=260)
Cold_coffee_label = tk.Label(bottom, text='Cold Coffee: ', font='arial 14 bold', bg='white')
Cold_coffee_label.place(x=30, y=340)
Tea_label = tk.Label(bottom, text='Tea: ', font='arial 14 bold', bg='white')
Tea_label.place(x=30, y=420)
Mineral_Water_label = tk.Label(bottom, text='Mineral Water: ', font='arial 14 bold', bg='white')
Mineral_Water_label.place(x=30, y=500)
Price_label = tk.Label(bottom, text='Price: ', font='arial 14 bold', bg='white')
Price_label.place(x=380, y=20)
vat_label = tk.Label(bottom, text='VAT: ', font='arial 14 bold', bg='white')
vat_label.place(x=380, y=100)
discount_label = tk.Label(bottom, text='Discount: ', font='arial 14 bold', bg='white')
discount_label.place(x=380, y=180)
total_price_label = tk.Label(bottom, text='Total Price: ', font='arial 14 bold', bg='white')
total_price_label.place(x=380, y=260)
button
btn = tk.Button(bottom, text='Count', font='arial 14 bold',bd=3, command=count_price)
btn.place(x= 130, y=580)
entry boxes
biriyani_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
biriyani_entry.insert(0,'0')
biriyani_entry.place(x=180, y=20)
kacchi_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
kacchi_entry.insert(0,'0')
kacchi_entry.place(x=180, y=100)
burger_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
burger_entry.insert(0,'0')
burger_entry.place(x=180, y=180)
coffee_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
coffee_entry.insert(0,'0')
coffee_entry.place(x=180, y=260)
cold_coffee_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
cold_coffee_entry.insert(0,'0')
cold_coffee_entry.place(x=180, y=340)
tea_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
tea_entry.insert(0,'0')
tea_entry.place(x=180, y=420)
mineral_water_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
mineral_water_entry.insert(0,'0')
mineral_water_entry.place(x=180, y=500)
price_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
price_entry.insert(0,'00 TAKA')
price_entry.place(x=500, y=20)
vat_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
vat_entry.insert(0,'00 TAKA')
vat_entry.place(x=500, y=100)
discount_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
discount_entry.insert(0,'00 TAKA')
discount_entry.place(x=500, y=180)
total_price_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
total_price_entry.insert(0,'00 TAKA')
total_price_entry.place(x=500, y=260)
So the full code is :-
import tkinter as tk
import datetime
from tkinter import messagebox
date1 = datetime.date.today()
win = tk.Tk()
win.title('Restaurant Management System')
win.geometry('850x700+300+5')
win.resizable(0,0)
# ------------------ food functions ------------------
def count_price():
biriyani_number = biriyani_entry.get()
kacchi_number= kacchi_entry.get()
burger_number = burger_entry.get()
coffee_number = coffee_entry.get()
cold_coffee_number = cold_coffee_entry.get()
tea_number = tea_entry.get()
mineral_water_number = mineral_water_entry.get()
try:
price_number = (int(biriyani_number)*230)+(int(kacchi_number)*260)+(int(burger_number)*190)+(int(coffee_number)*60)+(int(cold_coffee_number)*130)+(int(tea_number)*20)+(int(mineral_water_number)*15)
price_entry.delete(0,'end')
price_entry.insert(0, (str(price_number)+' '+'TAKA'))
vat_entry.delete(0,'end')
vat_entry.insert(0,'00 TAKA')
discount_entry.delete(0,'end')
discount_entry.insert(0,'00 TAKA')
total_price_entry.delete(0,'end')
total_price_entry.insert(0,(str(price_number)+' '+ 'TAKA'))
except:
tk.messagebox.showerror('Error', 'Please enter valid numbers in all the fields.')
# -------------------- frames -----------------
top = tk.Frame(win,height=150 ,bg='light blue')
top.pack(fill=tk.BOTH)
bottom = tk.Frame(win, height=500, bg='white')
bottom.pack(fill=tk.BOTH, expand=True)
# ------------------ heading ------------------
heading = tk.Label(top, text='Jashim Tea Stall and Restaurant', bg='light blue', font='arial 17 bold')
heading.pack()
today_date = tk.Label(top, text=f'Date: {date1}', bg='light blue', font='arial 14 bold')
today_date.pack()
# ------------------ labels --------------------
biriyani_label = tk.Label(bottom, text='Biriyani: ', font='arial 14 bold', bg='white')
biriyani_label.place(x=30, y=20)
kacchi_label = tk.Label(bottom, text='Kacchi: ', font='arial 14 bold', bg='white')
kacchi_label.place(x=30, y=100)
burger_label = tk.Label(bottom, text='Burger: ', font='arial 14 bold', bg='white')
burger_label.place(x=30, y=180)
coffee_label = tk.Label(bottom, text='Coffee: ', font='arial 14 bold', bg='white')
coffee_label.place(x=30, y=260)
Cold_coffee_label = tk.Label(bottom, text='Cold Coffee: ', font='arial 14 bold', bg='white')
Cold_coffee_label.place(x=30, y=340)
Tea_label = tk.Label(bottom, text='Tea: ', font='arial 14 bold', bg='white')
Tea_label.place(x=30, y=420)
Mineral_Water_label = tk.Label(bottom, text='Mineral Water: ', font='arial 14 bold', bg='white')
Mineral_Water_label.place(x=30, y=500)
Price_label = tk.Label(bottom, text='Price: ', font='arial 14 bold', bg='white')
Price_label.place(x=380, y=20)
vat_label = tk.Label(bottom, text='VAT: ', font='arial 14 bold', bg='white')
vat_label.place(x=380, y=100)
discount_label = tk.Label(bottom, text='Discount: ', font='arial 14 bold', bg='white')
discount_label.place(x=380, y=180)
total_price_label = tk.Label(bottom, text='Total Price: ', font='arial 14 bold', bg='white')
total_price_label.place(x=380, y=260)
# --------------------- btn ---------------
btn = tk.Button(bottom, text='Count', font='arial 14 bold',bd=3, command=count_price)
btn.place(x= 130, y=580)
# ----------------------- entry boxes--------------------
biriyani_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
biriyani_entry.insert(0,'0')
biriyani_entry.place(x=180, y=20)
kacchi_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
kacchi_entry.insert(0,'0')
kacchi_entry.place(x=180, y=100)
burger_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
burger_entry.insert(0,'0')
burger_entry.place(x=180, y=180)
coffee_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
coffee_entry.insert(0,'0')
coffee_entry.place(x=180, y=260)
cold_coffee_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
cold_coffee_entry.insert(0,'0')
cold_coffee_entry.place(x=180, y=340)
tea_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
tea_entry.insert(0,'0')
tea_entry.place(x=180, y=420)
mineral_water_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
mineral_water_entry.insert(0,'0')
mineral_water_entry.place(x=180, y=500)
price_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
price_entry.insert(0,'00 TAKA')
price_entry.place(x=500, y=20)
vat_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
vat_entry.insert(0,'00 TAKA')
vat_entry.place(x=500, y=100)
discount_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
discount_entry.insert(0,'00 TAKA')
discount_entry.place(x=500, y=180)
total_price_entry = tk.Entry(bottom, width=25, bd=5, font='arial 8 bold')
total_price_entry.insert(0,'00 TAKA')
total_price_entry.place(x=500, y=260)
Output
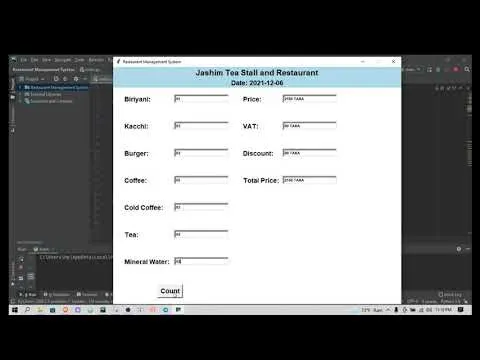