An Armstrong number is such a number which is equal to the sum of cube of its individual digit. For example 153, 370, 371 and so on are Armstrong number. I think this example will elucidate what is meant by Armstrong number. Lets take a number 370.
We can see:
370 = 33 + 73 + 03 = 27 + 343 + 0 = 370.
#Python program to check whether the user input number is Armstrong number or not
Input=int(input("Enter a number to check: "))
Sum=0
temp=Input
while temp > 0:
Number=temp%10
Cube=Number*Number*Number
Sum+=Cube
temp=temp//10
if Input==Sum:
print ("The entered number-{}, is an armstrong number.".format(Input))
else:
print("The entered number-{}, is not an armstrong number.".format(Input))
The output as executed by me is as follows"
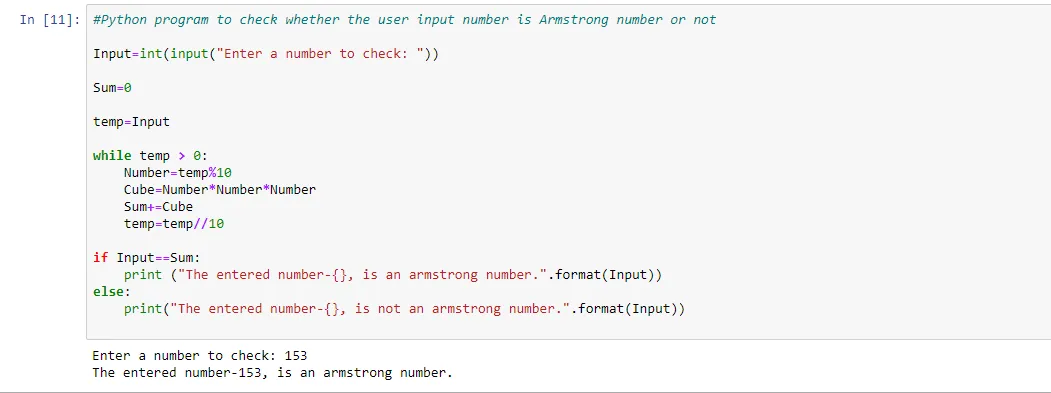
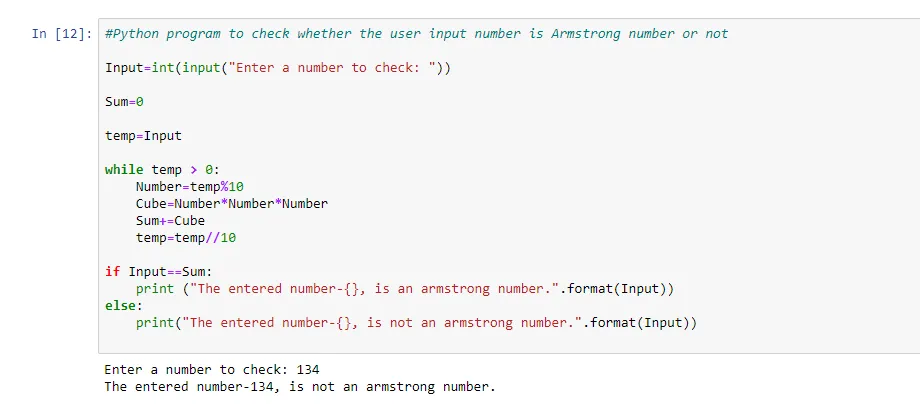
This is how loop in my code works for the number 153, which is an armstrong number.
temp=153
First Loop:
Number=153%10=3
Cube=3 * 3 * 3=27
Sum=0+27=27
temp=153/10=15(it calculates quotient only)
Since temp=15>0 the while loop again continues.
Second Loop:
Number=15%10=5
Cube=5 * 5 * 5=125
Sum=27+125=152
temp=15/10=1
Since temp=1 > 0, the while loop again iterates.
Third Loop:
Number=1%10=1
Cube=1 * 1 * 1 = 1
Sum=152+1=153
temp=1/10=0
Since temp =0, this doesn't satisfy our while loop condition and the loop halts.
The Sum =153 which is our input, so it gives output as being an Armstrong number.
Copy paste and run this code online here.