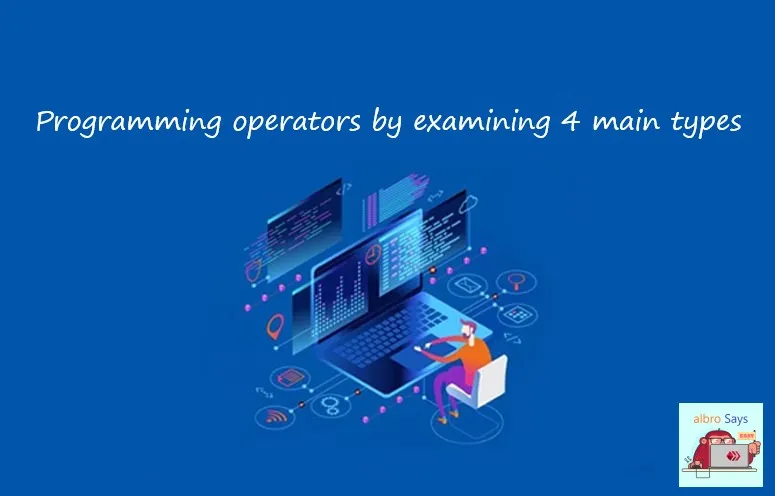
An operator in a programming language is a symbol that specifies a specific operation. This operation is usually a mathematical, relational or logical operation. In this post, we will learn about the concept of operator and types of programming operators so that we can use them properly in software development.
Operators exist in most programming languages. They are used to perform mathematical operations, comparing values or logical combinations.
Usually, the concept and symbol of basic operators are fixed in languages. Outside the world of programming, we have also dealt with operators; For example, mathematical operators (such as sum and multiplication).An operator is a symbol that has one or more operands. This symbol specifies what the compiler or interpreter of the programming language should do with the operands.
Types of operators in programming
In one sense, we can divide operators based on the number of their operands. But the more common and correct classification is dividing operators based on the type of work they do.
In this division, we have four types of operators in programming:
- Mathematical operator
- Relational operator
- Logical operator
- Bitwise operator
In the next sections, we will define each of these operators and get to know them.
Assignment Operator
A general operator that exists in all languages and does not fit into any of the above categories is the assignment operator. The assignment operator is represented as =
and has two operands.
One of the operands is placed on the right side of equality and its value is poured into the left operand. We use this operator to define the programming variable. You can also change the value of variables in the program with the assignment sign.
In the code below, in the first line, I have set the value of variable x
equal to 17, and in the second line, variable y
has been set to 25. In the third line, we add these two values together and put the result in a variable called result
.
x = 7
y = 25
result = x + y
Mathematical operators in programming
Calculations in computer programs are an inseparable component and operation. In the simplest case, we can write a program to add two numbers (similar to the third line of the code above) or create a very complex program to solve heavy math problems.
In any case, we must use mathematical operators in it to perform basic operations. You are definitely familiar with most of the mathematical operators from your school days. The operators of addition, subtraction, multiplication, division, power, etc.
Each of these operators is used with a symbol similar to what we have used so far in programming languages. In the table below, you can see the mathematical operators that accept two operands.
Symbol | Operation | Example |
---|---|---|
+ | Sum two values | 5 + 17 |
- | Subtracting two values | 29 - 7 |
* | Multiplication two operands | 11 * 7 |
/ | Dividing two values | 26 / 3 |
% | Correct division remainder | 34 % 5 |
** | Power first to second | 3 ** 4 |
We have rarely used the division remainder sign in everyday mathematics. Consider the same example given in the table. If we divide the number 34 by 5, our correct result is equal to 6 and 4 remains. In this case, we say that the remainder of 4 is the correct division of 34 by 5.
Each operand of an operator can be a specified value or a variable. As a result, we can add the value of two variables together or add a constant value to a variable. In the following sections, you will see more examples of variable operands.
Mathematical compound operators
In many languages, there are a series of compound mathematical operators for further simplification in programming. Suppose we have two variables x
and y
. We want to add these two together and replace the previous value in the x
variable.
The first thing that comes to mind is to write: x = x + y
This statement is absolutely correct. But if you look carefully, we have written the variable name x
twice. Math compound operators combine the math operator and the assignment operator to give us a new operator. With the help of these operators, instead of the above expression, we write: x += y
In the table below, you can see five compound operators.
Symbol | Operation | Example |
---|---|---|
+= | Assignment of sum | x += y |
-= | Assignment of subtracting | x -= 3 |
*= | Assignment of multiplication | x *= y |
/= | Assignment of dividing | x /= 5 |
%= | Assignment of correct division remainder | x %= 5 |
Increment or decrement math operator
It doesn't matter if you are at the beginning of programming or have years of experience, you always need to increase or decrease the values of some variables by one unit. Usually, more operations of this type are performed in repetition statements or other parts of the program where you need to count the number of repetitions.
So far we have learned two methods to increase a variable by one unit.
x = x + 1
x += 1
A shorter command to increment or decrement a variable value by one is to use the ++
or --
operators. (read plus plus or minus minus)
If such a sign is placed after the variable name, one unit is added or subtracted from its numerical value.
x++
x--
In some languages, we can also call this operator before the variable name; in such a way that we have --x
or ++x
.
Relational operators in programming
Other operators that we use a lot in programming are relational operators. As the name suggests, these operators are used to check the relationship between two values.
The relationship between two values is defined as greater than, less than or equal to. Basically we are comparing two values together. For this reason, these types of operators are also called comparison operators.
Suppose we have two numerical variables named a
and b
. We want to compare the values of these two. In mathematical symbols, we use =
for equality, >
for greater and <
for less.
We use the same signs for the relational operator with one difference: because the =
sign is used for the assignment operator, ==
is used to check the equality of two values.
Symbol | Discuss |
---|---|
== | Equality |
!= | Inequality |
> | Greater |
< | Less |
>= | Greater than or equal to |
<= | Less than or equal to |
We usually use these operators in conditional statements.
Logical Operators
Almost the most important operators in computer programming are logical operators. These operators are used to perform logical operations between different values.
Logical operation means AND
, OR
and NOT
operations. Usually, the operand of a logical operator is a logical value. But according to the definition of each data type in different languages, we can use other data types as well. Of course, this does not seem very logical and correct.
I mean logical data as true
and false
values.
You can see the symbols of three logical operations in the table below. The first two operators can be used repeatedly. For example, we can logically AND
together 4 values.
Symbol | Discuss |
---|---|
&& | Logical AND operation |
|| | Logical OR operation |
! | Logical NOT operation |
The not
operation is performed on an operand and its sign is placed before the name of the operand.
In the following table, the result of performing logical operations on two data with different values (true
or false
) is displayed.
A | B | A && B | A || B | !A |
---|---|---|---|---|
False | False | False | False | True |
False | True | False | True | True |
True | False | False | True | False |
True | True | True | True | False |
Bitwise operators
In some programming languages, operators are considered to perform operations on bits. The operands of bit operators are viewed as a string of 0
and 1
, or the binary basis.
For example, the result of executing and
between two values 0110
and 1010
will be 0010
.
Symbol | Discuss |
---|---|
& | bitwise and |
| | bitwise or |
~ | bitwise not |
In some programming languages, such as C, you are only allowed to use bitwise operators for single-byte values (such as character or int). But in some other languages, if you use bitwise operators between two non-binary operands, the value of those operands is converted into a bit string and then the operation is performed on them.
The important thing is that both operands in bitwise operators must have the same length.