Hello hive friends, for me it is a pleasure to be able to make one more publication in this beautiful community, what we are going to do is a "text to speed" we are going to write in a text field and when we press a button the computer will tell us what is written in the text field, without more than saying my name is José Sojo and I will leave you with this tutorial
Hola hive amigos, para mi es un placer poder hacer una publicación más en esta hermosa comunidad, lo que vamos a hacer es un "text to speed" vamos a escribir en un campo de texto y cuando precionemos un botón el computador nos va a decir lo que está escrito en el campo de texto, sin más que decir mi nombre es José Sojo y los dejo con este tutorial
We will do it in the Javascript (js) programming language so we will need:
Lo haremos en el lenguaje de programación Javascript (js) así que necesitaremos:
Browser: How the practice is in the javascript language and as javascrpit is native to the web we need a web browser to visualize the changes
Navegador: Cómo la práctica es en el lenguaje javascript y como javascrpit es nativo de la web necesitamos un navegador web para visualizar los cambios
Text editor: We will need a text editor in which we will write the necessary code for our practice to work, for example Sublime text, Visual Studio
Editor de texto: Necesitaremos un editor de text en el cual vamos a escribir el codigo necesario para que nuestra practica funcione, por ejemplo Sublime text, Visual Studio
Those and the desire to learn is all we need ...
Esos y las ganas de aprender es todo lo que necesitamos...
The tutorial was divided into two parts to be easier to understand, the first part is the structure and the next is the logic
El tutorial lo dividí en dos partes para ser más sencillo su entendimiento, la primera parte es la estructura y la siguiente es la lógica
We will design the structure in HTML, for the styles part we will use the "boostrap" style library to save the styles part, the html structure would be like this:
La estructura la diseñaremos en HTML, para la parte de los estilos utilizaremos la librería de estilos "boostrap" para ahorrarnos la parte de los estilos, la estrutura del html nos quedaría de esta forma:
The head: it is very simple is inside the head tags inside it has
the "meta" tag, with the "charset" attribute, with the value "UTF-8" which is for the encoding to put accents and special characters
the "title" tag that is for the title that goes in the browser tab
the "link" tag that links the "boostrap" styles that are locally
El head: es muy sencillo está dentro de las etiquetas head dentro tiene
la etiqueta "meta", con el atributo "charset", con el valor "UTF-8" que es para la codificación para poner colocar acentos y caracteres especiales
la etiqueta "title" que es para el titulo que va en la pestaña del navegador
la etiqueta "link" que enlaza los estilos "boostrap" que está de forma local
<head>
<meta charset="UTF-8">
<title>Text to speak</title>
<link rel="stylesheet" type="text/css" href="bootstrap.min.css">
</head>
The body: the body is very simple (all classes are of the boostrap styles) it only has:
a div that will contain all the fields and has the "boostrap" classes to make it look good
inside it has an input of type "text" with an id "text" in the same way it has the classes of "boostrap" so that it looks good
then we have a button, with the id "speak" and the bootrap classes so that it has a good appearance
At the end we have something very important is the "script" that links to the javascript code
Remember all styles are from boostrap
El body: el body es muy sencillo (todas las clases son de los estilos de boostrap) solo tiene:
un div que contendrá todos los campos y tiene las clases de "boostrap" para que se vea bien
dentro tiene un input de tipo "text" con un id "text" de igual forma tiene las clases de "boostrap" para que luzca bien
luego tenemos un button, con el id "speak" y las clases de bootrap para que tenga una buena apariencia
Al final tenemos algo muy importante es el "script" que enlaza al cógigo javascript
Recuerda todos los estilos son de boostrap
<body>
<div class="container p-5 bg-light shadow-lg mt-5 d-flex justify-content-around">
<input type="text" id="text" class="w-50 form-control">
<button id="speak" class="w-25 btn btn-primary">Speak</button>
</div>
<script src="main.js"></script>
</body>
Now let's make the Javascript code, the logic of our project
Ahora hagamos el código Javascript, la lógica de nuestro proyecto
First we select the button by the id "speak" that we have already declared, to that id we apply the event listener with its "click" event that will execute the "toSay" function that we will create later.
Primero seleccionamos el button por el id "speak" que ya se lo declaramos, a ese id le aplicamos la escucha de eventos con su evento "click" que ejecutará la función "toSay" que crearemos más adelante
document.getElementById('speak').addEventListener("click",()=>{
toSay(document.getElementById("text").value);
});
Now we create the function and store it in a constant because it is an arrow type function, which receives a parameter in this case is "textSpeak", now what will this function do? ... this function accesses the "speechSynthesis" object in its "speak" method that receives a parameter that is first we declare that it will be a new instance with the word "new" of "SpeechSynthesisUtterance" and we give it as a parameter "textSpeak" which is the same parameter that the function receives
Ahora creamos la función la almacenamos en una constante por que es una función de tipo flecha, que recibe un parámetro en este caso es "textSpeak", ahora ¿Que hará esta función?... esta función accede al objeto "speechSynthesis" en su método "speak" que recibe un parámetro que es primero declaramos que será una nueva instancia con la palabra "new" de "SpeechSynthesisUtterance" y le damos cómo parámetro "textSpeak" que es mismo parámetro que recibe la función
const toSay = (textSpeak)=>{
speechSynthesis.speak(new SpeechSynthesisUtterance(textSpeak));
}
All the code together would look like this ... first select the button and give it the click event that executes a function and that function executes the "toSay" function and we give it as a parameter the input selection and access its value
Todo el código junto se vería así... primero selecciona el botón y le da el evento click que ejecuta una función y esa función ejecuta la función "toSay" y la damos como parámetro la seleccion de input y accedemos a su valor
document.getElementById('speak').addEventListener("click",()=>{
toSay(document.getElementById("text").value);
});
const toSay = (textSpeak)=>{
speechSynthesis.speak(new SpeechSynthesisUtterance(textSpeak));
}
</code>
Well, that's all for now, it's a very simple practice, but it helps us increase our programming knowledge and it's very useful.
Y bueno eso es todo por ahora es una practica muy sencilla pero nos ayuda a aumentar nuestros conocimientos en la programación y es muy util
I hope you have been able to understand the tutorial and you can also do your practice ... Without more to say, I can only thank you for having read this far ... follow me so that you can continue to see my content, and here I say goodbye my name is José Sojo and see you next time
Espero hayas podido entender el tutorial y tu tambien puedas hacer tu practica... Sin más que decir solo me queda agradecerte por haber leído hasta aquí... sigueme para que puedas seguir viendo mi contenido, y aquí me despido mi nombre es José Sojo y nos vemos en la próxima
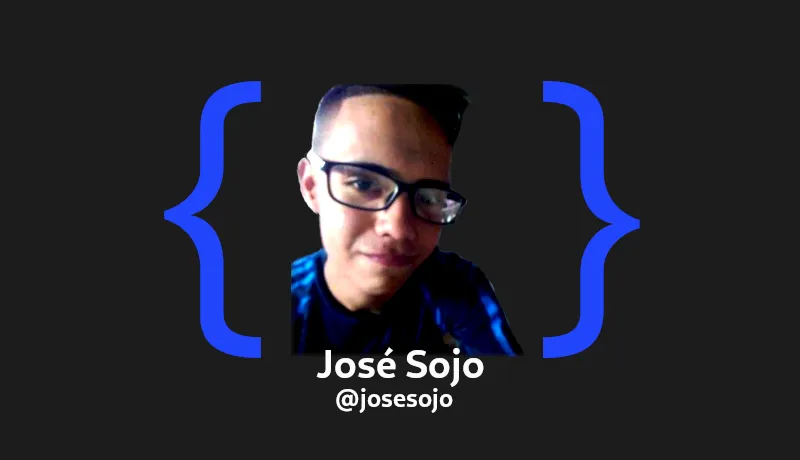