Hello Everyone
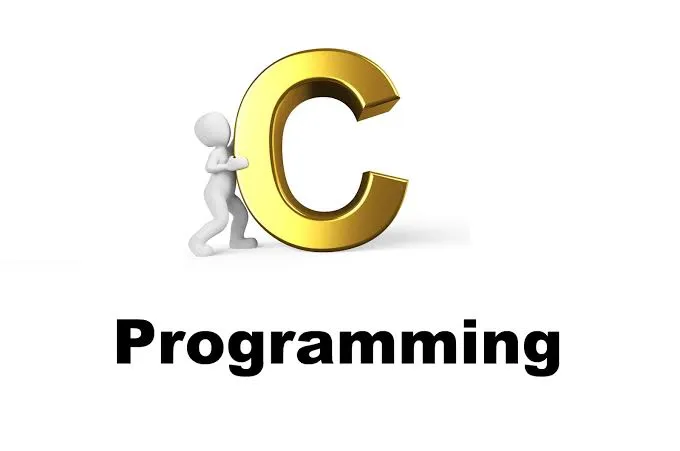
I hope you all are fine and safe inside your homes. This is a weird time ongoing in the whole world and I hope it will get over soon. As during this time, everyone is locked into their homes. I want to share the C programming language with you. I hope you guys like it, so let's happen today's topic.
Recursion
What is Recursion?
Recursion is the process of repeating items in a self-similar manner. Same is the case in programming if a program allows user to call a function inside the same function, then it is said to be Recursive call by function.
Syntax:
void recursion() {
recursion(); /* function calls itself */
}
int main() {
recursion();
}
C programming language supports Recursion which means a user can call a function inside the same function by itself. By using Recursion, programmers need to be careful to set an exit condition, otherwise, it will go into an infinite loop.
The benefit of Recursive functions is that they are very useful in solving many mathematical problems like calculating factorial, generating Fibonacci series, and many other.
- 1:Program for Number Factorial:
Factorial of a number can be found by using the program down below using Recursive function.
#include
int factorial(unsigned int i) {
if(i <= 1) {
return 1;
}
return i * factorial(i - 1);
}
int main() {
int i = 12;
printf("Factorial of %d is %d\n", i, factorial(i));
return 0;
}
After executing the above program, output will be:
Factorial of 12 is 479001600
- 2:Program for Fibonacci Series
Fibonacci series can also be generated by using Recursive function.
#include
int fibonacci(int i)
{
if(i == 0) {
return 0;
}
if(i == 1) {
return 1;
}
return fibonacci(i-1) + fibonacci(i-2);
}
int main() {
int i;
for (i = 0; i < 10; i++) {
printf("%d\t\n", fibonacci(i));
}
return 0;
}
After executing the above program, output will be:
0
1
1
2
3
5
8
13
21
34
- 3:Program for Binary Search:
Binary search can be also done by using Recursive function, here is the program for Binary Search:
#include
void binary_search(int [], int,int, int);
void bubble_sort(int [], int);
int main()
{
int key, size, i;
int list[25];
printf("Enter size of a list: ");
scanf("%d", &size);
printf("Generating random numbers\n");
for(i = 0; i < size; i++)
{
list[i] = rand() % 100;
printf("%d ", list[i]);
}
bubble_sort(list, size);
printf("\n\n");
printf("Enter key to search\n");
scanf("%d", &key);
binary_search(list, 0, size, key);
}
void bubble_sort(int list[], int size)
{
int temp, i, j;
for (i = 0; i < size; i++)
{
for (j = i; j < size; j++)
{
if (list[i] > list[j])
{
temp = list[i];
list[i] = list[j];
list[j] = temp;
}
}
}
}
void binary_search(int list[], int lo, int hi, int key)
{
int mid;
if (lo > hi)
{
printf("Key not found\n");
return;
}
mid = (lo + hi) / 2;
if (list[mid] == key)
{
printf("Key found\n");
}
else if (list[mid] > key)
{
binary_search(list, lo, mid - 1, key);
}
else if (list[mid] < key)
{
binary_search(list, mid + 1, hi, key);
}
}}
Output:
Enter size of a list: 10
Generating random numbers
83 86 77 15 93 35 86 92 49 21
Enter key to search
21
Key found

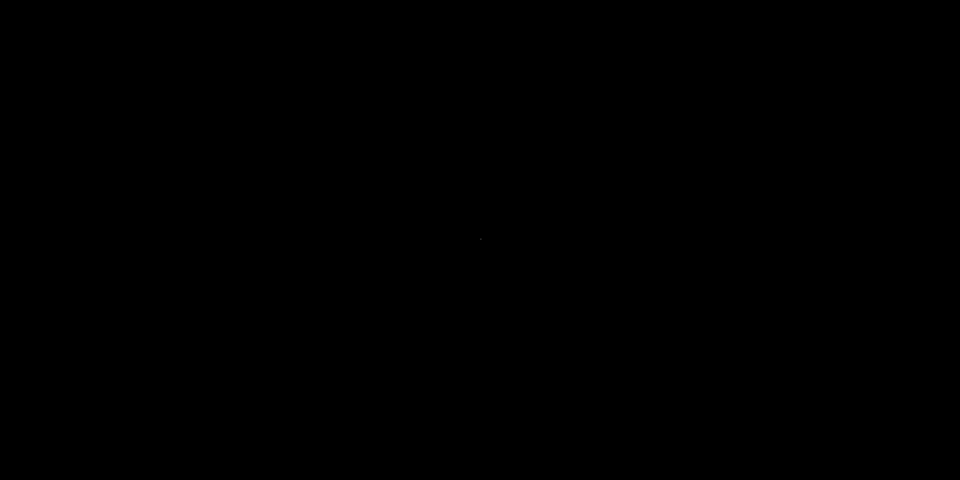

Thank you.
I hope you guys liked my post.
Keep Supporting.
STAY TUNED FOR NEXT POST
UPVOTE COMMENT RESTEEM
IF YOU LIKED MY POST

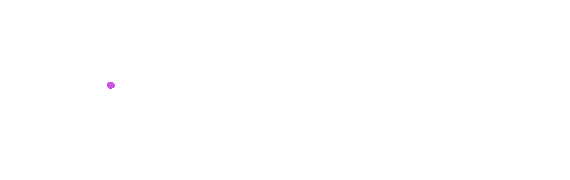
Stay Home, Stay Safe
@peerzadazeeshan
PEACE✌️✌️
I hope you guys liked my post.
Keep Supporting.
STAY TUNED FOR NEXT POST
UPVOTE | COMMENT | RESTEEM |
---|---|---|
IF YOU | LIKED | MY POST |

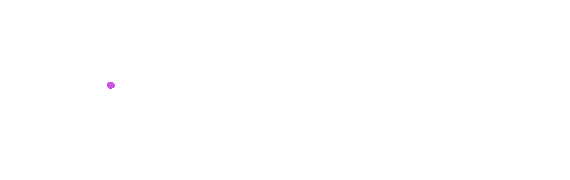